Delving into the world of computer programming can be both exciting and challenging. One engaging way to practice your C programming skills is by creating pattern programs. These programs use loops and control flow statements to generate interesting designs on the console screen, often using characters like asterisks (*), numbers, or alphabets. They provide a fun and visual way to solidify your grasp of fundamental programming concepts.
Table of contant
Basics of C Programming
Putting It All Together: Fundamentals of C Programming
You may write reliable software applications by learning C programming, which is a fundamental language in the field. It’s important to confirm that you understand the fundamentals before taking on complicated assignments. The essential components of C programming are broken down as follows:
Syntax and Structure: Like every other language, C has its own set of guidelines for writing code. This involves using keywords (words reserved for certain meanings), operators (symbols used for calculations and comparisons), and punctuation correctly (semicolons, commas, etc.). Knowing syntax helps you write code that is both computer-readable and grammatically sound.
Variables and Data Types: In your program, variables serve as storage spaces for data. You can declare variables in C with several data types, indicating the kinds of information that they can store. Characters (single letters), strings (character sequences), integers (whole numbers), and floating-point numbers (decimals) are examples of common data types. Using the right data types when declaring variables helps you avoid errors and maintain organization in your code.
Control statements (switch, if-else):
The program’s execution flow is determined by these statements. They let you base decisions on specific circumstances. You can run separate code blocks based on whether a condition is true or false using the if-else statement. Several conditions and related actions can be handled more succinctly with the switch statement. Through control statement proficiency.
For, while, and do-while loops are strong programming constructs that are used to repeat a block of code until a predetermined number of times or until a specified condition is met.
C provides three basic forms of loops:
For loop: Usually used in conjunction with a counter variable, this expression runs a block of code a predefined number of times.
While loop: Runs a code block repeatedly as long as a predetermined condition holds true.
Do-while loop: This loop functions similarly to a while loop, except even in cases where the condition is initially false, the code block runs at least once.
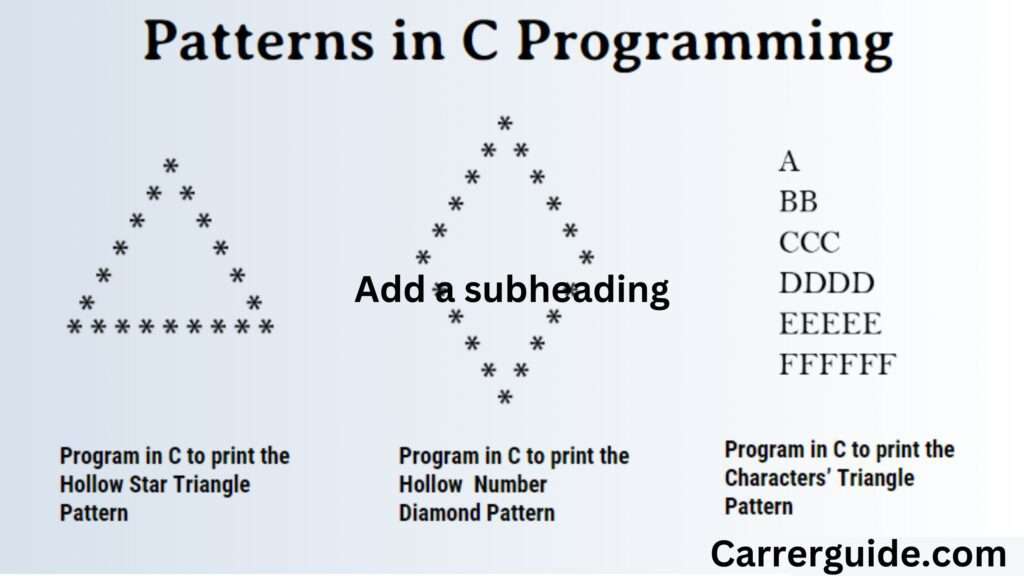
Pattern Programs Using Loops
Creating Eye-Cracking Images: Creating Pattern Programs with C Loops
After learning the foundations of C programming, let’s explore the fascinating world of pattern programs. These applications leverage characters such as asterisks (*), integers, or alphabets to create visually appealing graphics on the console screen using loops. They help you grasp loops and control flow more thoroughly while also adding fun to the process of learning C.
Basics of Looping: Three main loop constructions are provided by A Refresher C, each with advantages of its own:
A code block can be run a preset number of times with a for loop. Usually, it makes use of a counter variable that is increased or decreased during the loop.
for (int i = 1; i <= 5; i++) { // Code to be repeated 5 times }
while loop:
Continues executing a code block as long as a specified condition remains true. This loop is useful for situations where the number of repetitions isn’t predetermined.
int i = 1;
while (i <= 5) {
// Code to be repeated
i++; // Increment counter to eventually exit the loop
}
do-while loop: Similar to a while
loop, but the code block executes at least once, even if the condition is initially false.
int i = 1;
do {
// Code to be repeated at least once
i++; // Increment counter for subsequent iterations
} while (i <= 5);
The Power of Nested Loops
Pattern programs often leverage the concept of nested loops. A nested loop places one loop entirely within another loop’s body. The inner loop executes completely for each iteration of the outer loop. This nesting allows for creating intricate and diverse patterns.
Here’s a simplified example:
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 4; j++) {
printf("*"); // Print an asterisk for each inner loop iteration
}
printf("\n"); // Move to the next line after each outer loop iteration
}
A Collection of Patterns: Typical Instances and Algorithms
C pattern programs cover a wide range of designs and are quite expansive. Here are a few well-known instances to pique your interest:
Number Pyramid: To print a pyramid of numbers in either ascending or descending order, use stacked loops.
Asterisk Pyramid: This pyramid construction is built using asterisks (*), just like the number pyramid.
Right-Angled Triangle: Make use of nested loops and asterisks (*) to form a triangle pattern.
Hollow Square/Rectangle: Create a pattern in the shape of a square or rectangle with asterisks (*) at the corners and empty spaces in the middle.
Simple Patterns Program in C
Basic C Pattern Programs: The Foundation of Beauty
Let’s start our study of pattern programming in C with some basic examples that demonstrate the usefulness of loops. You can practice fundamental formatting techniques and strengthen your understanding of loop constructions with the aid of these applications.
1. Printing a Line of Stars:
This program shows how to print a specified number of asterisks (*) on a single line using a basic for loop.
#include <stdio.h>
int main() {
int numStars;
printf("Enter the number of stars to print: ");
scanf("%d", &numStars);
// Loop to print stars
for (int i = 1; i <= numStars; i++) {
printf("*");
}
printf("\n"); // Add a newline character at the end
return 0;
}
2. Printing Numbers in a Line:
This program utilizes a for
loop to print a sequence of numbers in a single line, either in ascending or descending order based on user input.
#include <stdio.h>
int main() {
int start, end;
char order;
printf("Enter the starting number: ");
scanf("%d", &start);
printf("Enter the ending number: ");
scanf("%d", &end);
printf("Enter 'a' for ascending or 'd' for descending: ");
scanf(" %c", &order); // Use space before %c to consume newline from previous input
if (order == 'a') {
// Ascending order
for (int i = start; i <= end; i++) {
printf("%d ", i);
}
} else if (order == 'd') {
// Descending order
for (int i = start; i >= end; i--) {
printf("%d ", i);
}
} else {
printf("Invalid order entered.\n");
}
printf("\n"); // Add a newline character at the end
return 0;
}
3.Printing Alphabets in a Line:
This program employs a for
loop to print all uppercase or lowercase alphabets in a single line, based on user preference.
#include <stdio.h>
int main() {
char startingChar, endingChar;
printf("Enter the starting alphabet (uppercase or lowercase): ");
scanf(" %c", &startingChar); // Use space before %c to consume newline from previous input
printf("Enter the ending alphabet (uppercase or lowercase): ");
scanf(" %c", &endingChar);
// Check if alphabets are in the same case (uppercase or lowercase)
if (startingChar >= 'a' && endingChar <= 'z' || startingChar >= 'A' && endingChar <= 'Z') {
// Print in ascending order
for (char c = startingChar; c <= endingChar; c++) {
printf("%c ", c);
}
} else {
printf("Alphabets must be in the same case (uppercase or lowercase).\n");
}
printf("\n"); // Add a newline character at the end
return 0;
}
Triangle Pattern Program in C
Expanding on the Details: Triangle Designs with Nesting Loops
Let’s explore the world of triangle patterns now that you have a firm grasp of basic patterns. These programs use asterisks (*) or other characters to make different triangle shapes using stacked loops.
1. Triangle with a right angle:
This program shows you how to use nested loops to generate a right-angled triangle. The inner loop prints the asterisks (*) in each row, while the outer loop determines how many rows there are.
#include <stdio.h>
int main() {
int rows;
printf("Enter the number of rows for the triangle: ");
scanf("%d", &rows);
for (int i = 1; i <= rows; i++) {
for (int j = 1; j <= i; j++) {
printf("*");
}
printf("\n"); // Move to the next line after each row
}
return 0;
}
2. Inverted Right-Angled Triangle:
This program utilizes nested loops to print an inverted right-angled triangle using asterisks (*).
#include <stdio.h>
int main() {
int rows;
printf("Enter the number of rows for the inverted triangle: ");
scanf("%d", &rows);
for (int i = rows; i >= 1; i--) {
for (int j = 1; j <= i; j++) {
printf("*");
}
printf("\n"); // Move to the next line after each row
}
return 0;
}
3. Equilateral Triangle:
An equilateral triangle has all sides equal in length. This program creates an equilateral triangle using nested loops and calculates the number of asterisks (*) to print in each row based on the total number of rows.
#include <stdio.h>
int main() {
int rows;
printf("Enter the number of rows for the equilateral triangle: ");
scanf("%d", &rows);
// Calculate the number of spaces for proper alignment
int spaces = rows - 1;
for (int i = 1; i <= rows; i++) {
// Print spaces for proper alignment
for (int j = 1; j <= spaces; j++) {
printf(" ");
}
// Print asterisks (*) for the current row
for (int k = 1; k <= 2 * i - 1; k++) {
printf("*");
}
printf("\n"); // Move to the next line after each row
spaces--; // Reduce spaces for subsequent rows
}
return 0;
}
4. Inverted Equilateral Triangle:
This program employs nested loops to generate an inverted equilateral triangle pattern using asterisks (*).
#include <stdio.h>
int main() {
int rows;
printf("Enter the number of rows for the inverted equilateral triangle: ");
scanf("%d", &rows);
for (int i = rows; i >= 1; i--) {
// Print spaces for proper alignment
for (int j = 1; j <= rows - i; j++) {
printf(" ");
}
// Print asterisks (*) for the current row
for (int k = 1; k <= 2 * i - 1; k++) {
printf("*");
}
printf("\n"); // Move to the next line after each row
}
return 0;
}
Square and Rectangle Pattern Program in C
Making Squares and Rectangles in C Using Loops
We now move on to the study of pattern programs using rectangles and squares. These patterns make forms with asterisks (*) or other characters, either filled or hollow in the middle, by using stacked loops.
1. Square Solid:
This application shows you how to use stacked loops to produce a solid square. The inner loop prints asterisks (*) in each row to create the square, while the outer loop determines how many rows are printed.
#include <stdio.h>
int main() {
int sideLength;
printf("Enter the side length of the square: ");
scanf("%d", &sideLength);
for (int i = 1; i <= sideLength; i++) {
for (int j = 1; j <= sideLength; j++) {
printf("*");
}
printf("\n"); // Move to the next line after each row
}
return 0;
}
2. Hollow Square:
This program creates a hollow square using nested loops. The outer loop controls the rows, and the inner loop conditionally prints asterisks (*) only on the borders of the square, leaving the center empty.
#include <stdio.h>
int main() {
int sideLength;
printf("Enter the side length of the hollow square: ");
scanf("%d", &sideLength);
for (int i = 1; i <= sideLength; i++) {
for (int j = 1; j <= sideLength; j++) {
// Print asterisk (*) only on the border (first and last column or first and last row)
if (i == 1 || i == sideLength || j == 1 || j == sideLength) {
printf("*");
} else {
printf(" "); // Print space for the hollow center
}
}
printf("\n"); // Move to the next line after each row
}
return 0;
}
3. Solid Rectangle:
This program utilizes nested loops to create a solid rectangle using asterisks (). Similar to the solid square, the outer loop controls the rows, and the inner loop prints asterisks () to form the rectangle.
#include <stdio.h>
int main() {
int rows, columns;
printf("Enter the number of rows for the rectangle: ");
scanf("%d", &rows);
printf("Enter the number of columns for the rectangle: ");
scanf("%d", &columns);
for (int i = 1; i <= rows; i++) {
for (int j = 1; j <= columns; j++) {
printf("*");
}
printf("\n"); // Move to the next line after each row
}
return 0;
}
4. Hollow Rectangle:
This program employs nested loops to generate a hollow rectangle pattern using asterisks (*). The concept is similar to the hollow square, but with separate loop conditions for rows and columns to create the hollow center.
#include <stdio.h>
int main() {
int rows, columns;
printf("Enter the number of rows for the hollow rectangle: ");
scanf("%d", &rows);
printf("Enter the number of columns for the hollow rectangle: ");
scanf("%d", &columns);
for (int i = 1; i <= rows; i++) {
for (int j = 1; j <= columns; j++) {
// Print asterisk (*) only on the border (first and last column or first and last row)
if (i == 1 || i == rows || j == 1 || j == columns) {
printf("*");
} else {
printf(" "); // Print space for the hollow center
}
}
printf("\n"); // Move to the next line after each row
}
return 0;
}
Pyramid Pattern Program in C
New Heights Achieved: C Pyramid Pattern Programs
Let’s investigate pyramid patterns as we move up the pattern programming ladder. These programs use asterisks (*) or other characters along with nested loops to build visually pleasing structures that resemble pyramids.
1. Basic Pyramid
This program shows you how to use nested loops to form a right-angled pyramid. The inner loop prints spaces for correct alignment and asterisks (*) to construct the pyramid, while the outer loop sets the number of rows.
#include <stdio.h>
int main() {
int rows;
printf("Enter the number of rows for the pyramid: ");
scanf("%d", &rows);
for (int i = 1; i <= rows; i++) {
// Print spaces for proper alignment
for (int j = 1; j <= rows - i; j++) {
printf(" ");
}
// Print asterisks (*) for the current row
for (int k = 1; k <= 2 * i - 1; k++) {
printf("*");
}
printf("\n"); // Move to the next line after each row
}
return 0;
}
2. Inverted Pyramid:
This program utilizes nested loops to generate an inverted pyramid pattern using asterisks (*). The logic is similar to the simple pyramid, but the loops iterate in a different order to achieve the inverted structure.
#include <stdio.h>
int main() {
int rows;
printf("Enter the number of rows for the inverted pyramid: ");
scanf("%d", &rows);
for (int i = rows; i >= 1; i--) {
// Print spaces for proper alignment
for (int j = 1; j <= rows - i; j++) {
printf(" ");
}
// Print asterisks (*) for the current row
for (int k = 1; k <= 2 * i - 1; k++) {
printf("*");
}
printf("\n"); // Move to the next line after each row
}
return 0;
}
3. Full Pyramid:
A full pyramid has a triangular structure on both sides. This program creates a full pyramid using nested loops. It combines the concepts of simple and inverted pyramids, with additional loops to build the left and right halves.
#include <stdio.h>
int main() {
int rows;
printf("Enter the number of rows for the full pyramid: ");
scanf("%d", &rows);
// Print upper half (simple pyramid)
for (int i = 1; i <= rows; i++) {
// Print spaces for proper alignment
for (int j = 1; j <= rows - i; j++) {
printf(" ");
}
// Print asterisks (*) for the current row
for (int k = 1; k <= 2 * i - 1; k++) {
printf("*");
}
printf("\n"); // Move to the next line after each row
}
// Print lower half (inverted pyramid) - adjust loop starting point and condition
for (int i = rows - 1; i >= 1; i--) {
// Print spaces for proper alignment
for (int j = 1; j <= rows - i; j++) {
printf(" ");
}
// Print asterisks (*) for the current row
for (int k = 1; k <= 2 * i - 1; k++) {
printf("*");
}
printf("\n"); // Move to the next line after each row
}
return 0;
}
4. Inverted Full Pyramid:
This program employs nested loops to generate an inverted full pyramid pattern using asterisks (*). Similar to the full pyramid, it combines the logic of inverted and simple pyramids, building the upper and lower halves.
#include <stdio.h>
int main() {
int rows;
printf("Enter the number of rows for the inverted full pyramid: ");
scanf("%d", &rows);
// Print upper half (inverted pyramid)
for (int i = rows; i >= 1; i--) {
// Print spaces for proper alignment
for (int j = 1; j <= rows - i; j++) {
printf(" ");
}
// Print asterisks (*) for the current row
for (int k = 1; k <= 2 * i
Alphabet Pattern Program in C
Getting Higher on the Alphabet Ladder: Symbol Programs
Let’s explore the realm of C’s letter patterns. These applications use character manipulation and nested loops to produce visually beautiful letter designs.
1. The Alphabet Triangle
This application shows you how to use uppercase alphabets to make a right-angled triangular pattern, starting at the top with the letter ‘A’ and working your way down.
#include <stdio.h>
int main() {
int rows;
printf("Enter the number of rows for the alphabet triangle: ");
scanf("%d", &rows);
char alphabet = 'A';
for (int i = 1; i <= rows; i++) {
// Print alphabets in ascending order for each row
for (int j = 1; j <= i; j++) {
printf("%c ", alphabet);
alphabet++; // Increment alphabet for the next character
}
printf("\n"); // Move to the next line after each row
}
return 0;
}
2. Alphabet Pyramid:
This program utilizes nested loops to generate an alphabet pyramid pattern. Similar to the alphabet triangle, it starts with ‘A’ at the top and progresses downwards, but also includes a lower half with alphabets in descending order.
#include <stdio.h>
int main() {
int rows;
printf("Enter the number of rows for the alphabet pyramid: ");
scanf("%d", &rows);
char alphabet = 'A';
// Print upper half (ascending order)
for (int i = 1; i <= rows; i++) {
// Print spaces for proper alignment
for (int j = 1; j <= rows - i; j++) {
printf(" ");
}
// Print alphabets in ascending order for the current row
for (int k = 1; k <= 2 * i - 1; k++) {
printf("%c ", alphabet);
alphabet++; // Increment alphabet for the next character
}
printf("\n"); // Move to the next line after each row
}
alphabet--; // Decrement alphabet to start from the last character for the lower half
// Print lower half (descending order) - adjust loop condition and alphabet manipulation
for (int i = rows - 1; i >= 1; i--) {
// Print spaces for proper alignment
for (int j = 1; j <= rows - i; j++) {
printf(" ");
}
// Print alphabets in descending order for the current row
for (int k = 1; k <= 2 * i - 1; k++) {
printf("%c ", alphabet);
alphabet--; // Decrement alphabet for the next character
}
printf("\n"); // Move to the next line after each row
}
return 0;
}
3. Alphabet Diamond:
A diamond pattern has a symmetrical structure with a point at the top and bottom. This program creates an alphabet diamond using nested loops. It combines the concepts of ascending and descending alphabet sequences to form the diamond shape.
#include <stdio.h>
int main() {
int rows;
printf("Enter the number of rows for the alphabet diamond: ");
scanf("%d", &rows);
char alphabet = 'A';
// Print upper half (ascending order)
for (int i = 1; i <= rows; i++) {
// Print spaces for proper alignment
for (int j = 1; j <= rows - i; j++) {
printf(" ");
}
// Print alphabets in ascending order for the current row
for (int k = 1; k <= 2 * i - 1; k++) {
printf("%c ", alphabet);
alphabet++; // Increment alphabet for the next character
}
printf("\n"); // Move to the next line after each row
}
alphabet -= 2; // Decrement alphabet twice to start from the second last character for the lower half
// Print lower half (descending order) - adjust loop condition and alphabet manipulation
for (int i = rows - 1; i >= 1; i--) {
// Print spaces for proper alignment
for (int j = 1; j <= rows - i; j++) {
printf(" ");
}
// Print alphabets in descending order for the current row
for (int k = 1; k <= 2 * i - 1; k++) {
Conclusion: Pattern Program in C
The Magical World of C Pattern Programs: A Summary
You have now embarked upon an engrossing voyage into the world of C pattern programs thanks to this exploration. You now know how to use control flow statements and loops to create visually appealing console screen designs.
Important Ideas Addressed:
Comprehending Loops (for, while, do-while): You have realized the ability of loops to run code blocks repeatedly or until a predetermined condition is satisfied.
Nested Loops: You learned how to build sophisticated structures by enclosing one loop completely inside the body of another loop, which allowed you to design intricate patterns.
Examples of Basic Patterns: You worked on creating basic patterns, such as printing alphabets, numbers, and stars in a row, which served as the basis for more complex designs.
Triangle Patterns: Using nested loops and computations for correct alignment, you learned how to make right-angled, inverted, equilateral, and inverted equilateral triangles.
Patterns for Squares and Rectangles: You experimented with making solid and hollow squares and rectangles, learning how to work with loops to adjust where the asterisks (*) go to create the desired forms.
Pyramid Patterns: By combining the ideas of triangle and loop manipulation, you dabbled in creating basic, inverted, full, and inverted full pyramids.
Alphabet Patterns: Using nested loops and character manipulation techniques, you ventured into the world of characters to create alphabet triangle, pyramid, and diamond patterns.
Beyond the Fundamentals:
These courses act as a launching pad for your artistic investigation. Please feel free to play around with these examples, alter them to make variations, and come up with original patterns of your own.
- To create patterns, use characters other than asterisks (*).
- Use ANSI escape sequences (for terminals that support them) to introduce color.
To make designs that are more intricate, combine different motifs. - Investigate user input to enable customization of characters, pattern size, and other features.
FAQ's: Pattern Program in C
Q1: What are pattern programs in C?
Ans:
Pattern programs are exercises that involve printing various patterns (such as stars, numbers, and alphabets) using loops in C programming. They are commonly used to practice and understand control flow, especially loops and nested loops.
Q2. Why should I learn pattern programs?
Ans.
Learning pattern programs helps improve logical thinking, understand loop control, and develop problem-solving skills. They are a fundamental part of mastering C programming.
Q3. What are the key concepts needed to write pattern programs?
Ans.
Key concepts include loops (for, while, do-while), conditional statements (if-else, switch), and nested loops.